Input
Input
Component
Lumberyard uses a unique system to get input data from a variety of controllers, including mouse and keyboard, and bind it to an event through its Input component. The component in turn references a .inputbinding file that actually defines the list of valid inputs that can then be referenced from a script to describe the behavior of every input. The Input component should be attached to the same entity as the script which is going to call the input event.
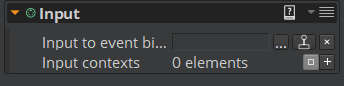
Input Binding
The .inputbinding file contains an Input Event Group. There can be multiple events in the group. Each event has its own event name and generators. Each event can have multiple generators, which means a certain event can be controlled by one or more inputs. The .inputbinding file can be added and edited in the Asset Editor. If the Asset Editor panel isn’t wide enough the plus/delete/clear buttons can be hidden, simply expand the panel if there are missing buttons of the right.
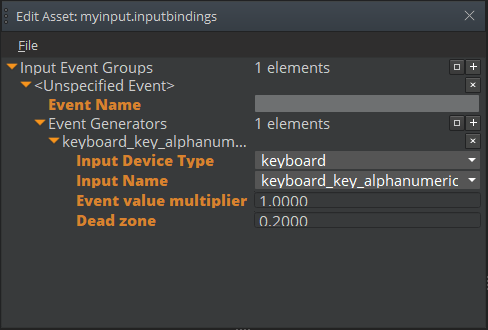
Scripting Interface
There are two ways to script the input:
1. Input Bus
Lumberyard doesn’t check input within an update loop, rather input events are subscribed to. When an input event occurs, the input notification bus is notified and any script listening for those event receives callbacks such as OnPressed, OnHeld, and OnReleased.
The script should be attached to the entity which has the input component. In scripting, the input component is connected with the scripts through the Event Name and the event value multiplier. The value is related to the input and is updated every frame. Using the values and the event name helps detect user input event that can be used to the trigger a reaction.
self.forwardBackInputBusId = InputEventNotificationId("NavForwardBack");
self.forwardBackInputBus = InputEventNotificationBus.Connect(self, self.forwardBackInputBusId);
There are three input functions: OnPressed(float); OnHeld(float); OnReleased(float); These functions can help us read the input events. In each events, we can check the value of the CurrentBusId and implement related reactions.
if (InputEventNotificationBus.GetCurrentBusId() == self.forwardBackInputBusId) then
-- do something
end
2.Gameplay Notification Bus
Another way to listen for input events is to use the StartingPointInput Gem, which provides Lua scripts that convert input bus events into gameplay bus events (held, pressed, and released scripts). Then in the script that recognizes input, rather than connecting to the input bus it connects to the gameplay bus. The reason to do this is that it allows for controls to be disabled by disabling the StartingPointInput script rather than updating a script, as well as helps with quick recognition of what input a entity is listening to. The downside to this method is that there is an additional string for the gameplay event that has the possibility of being mistyped.
self.ForwardBackId = GameplayNotificationId(self.entityId, "NavForwardBack", "float");
self.ForwardBack = GameplayNotificationBus.Connect(self.entityId, self.forwardBackId);
function script1:OnEventBegin(value)
-- do something
end
function script1:OnEventUpdating(value)
-- do something
end
function script1:OnEventEnd(value)
-- do something
end
This functionality is contained within the StartingPointInputGem, however can easily be scripted.
function script2:OnPressed(floatValue)
GameplayNotificationBus.Event.OnEventBegin(GameplayNotificationId(self.entityId, "NavForwardBack", "float"), floatValue)
end
function script2:OnHeld(floatValue)
GameplayNotificationBus.Event.OnEventUpdating(GameplayNotificationId(self.entityId, "NavForwardBack", "float"), floatValue)
end
function script2:OnReleased(floatValue)
GameplayNotificationBus.Event.OnEventEnd(GameplayNotificationId(self.entityId, "NavForwardBack", "float"), floatValue)
end